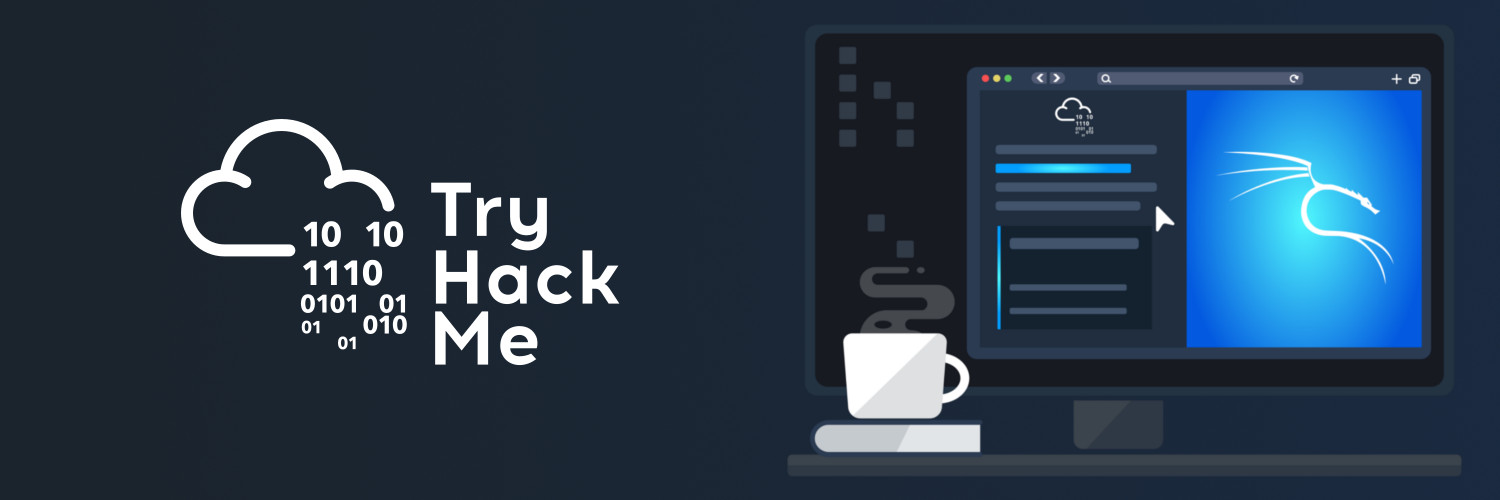
I have been a TryHackMe fanboy for a while and just finished up doing a 500 day streak and have completed 275+ rooms. I wanted to write a blog post describing my personal ten favorite rooms.
No flags or hints on how to complete these rooms on this page. I will discuss at a high level what the room is about and what skills or techniques would be needed to solve it. If you like to go into rooms 100% blind than feel free to skip this blog post.
10. Vulnversity

Vulnversity is a free and beginner friendly TryHackMe Room that is geared towards teaching the basics of Pentesting.
The room includes a video guide by Darkstar and does a fantastic job of explaining the tools used, thought processes, and holds your hand throughout the room. This is great for someone that is looking for their first penetration testing room that wants their hand held from start to finish.
This was one of the first rooms I did when I started TryHackMe and it cemented a number of techniques and tools I still use today. Port scanning, file and directory fuzzing, using reverse shells, and privilege escalation. Often I get asked what a good starting room is for penetration testing. This is the one I recommend.
Tools/Techniques that you could use are
- Nmap
- GoBuster
- Burp
- Netcat
- Using Linux to abuse SUID to become root
9. Jason

Who doesn’t love a good horror movie? Jason is a room that is fairly easy, but the technique used to complete the room can be a bit complicated for those new to penetration testing.
It is an easy room if you know what deserialization vulnerabilities are and how to exploit them. If this is something you are not familiar with then here is an OWASP article for you to read if you are interested.
If you want to practice deserialization then this room is for you. It is one of the few rooms that if you are struggling with deserialization I recommend using a guide or walk through to help get you through and learn about the concept.
Great room with fun challenges, great design, and helps in learning a rather daunting technique. Highly recommend checking this out if you are on the hunt to learn more about exploiting deserialization vulnerabilities.
8. Thompson

Thompson has a special place in my heart. I used to manage Apache Tomcat and this room is all about exploiting Tomcat in a fun and real world scenario.
I love when a box has a real world feel to it and Thompson is a scenario that penetration testers will see in the wild and have an opportunity to exploit. Tomcat is deployed with multiple default configurations and files that attackers will use against the target.
From the starting point of the room you get to have a lot of fun fuzzing for files and directories, password spraying, and using Metasploit to exploit Tomcat Manager to get into the system with a reverse shell.
I have seen this similar room used in competitions and it is definitely a room you want to check out.
Tools/Techniques that you could use are
- Nmap
- GoBuster
- Hydra
- Metasploit
- Abusing crontab for privilege escalation
7. Linux Agency

This box is 100% a video game box. It is a fun and exciting way to learn more about Linux commands.
The premise is: you start off on mission one. You ssh into the box as mission1 and are given a task to solve that allows you to become mission2 user and you essentially climb a ladder solving a challenge and then becoming a new user to solve another challenge.
The challenges change from different Linux techniques such as outputting data from a file, grepping a flag out of a file that has thousands of lines of data in it, changing file permissions, compiling files to get output and using various programming languages to read files.
Linux Agency is fun. However it is long so be prepared to spend some time on this room, but when it is done you will level up your Linux knowledge.
6. Wreath

Wreath is a large room aimed at teaching pivoting and Command and Control Frameworks. It is a very large room that can eat up a day.
It is highly recommend to take good notes and take it slow. I swear I didn’t plan this but again Dark has created a video guide to help teach and walk users through this if needed. The video guide is forty one videos and as stated earlier this can easily eat a whole day.
That being said it is a “free” room. The catch is it is free if the free user has a seven day streak.
This room goes into great detail on pivoting and what it is and with socat, chisel, and SSH Tunneling / Port Forwarding. The next major section will be Command and Control. If you are not familiar, Command and Control (C2 Frameworks) are applications used to manage remote sessions on a compromised host. Cobalt Strike, Covenant, Metasploit, and Empire are examples of C2 Frameworks. The last major section is AV Evasion.
I suggest this beast of a room because it teaches excellent fundamentals for internal pentesting and how to pivot and use C2 Frameworks. I cannot even remotely begin to explain how cool and amazing this room is. You will gain so much hands on experience and it is free for users with a seven day streak. I would easily pay money to TryHackMe to have access to this room.
5. Buffer Overflow Prep

Before I started TryHackMe, I had some basic Ethical Hacking Knowledge. I knew how to do a lot of the easy rooms and felt comfortable with using tools such as Nmap, Metasploit, GoBuster, Burp, etc. What I didn’t feel comfortable with was Buffer Overflows.
Buffer Overflow Prep by Tib3rius was a God send for me. It taught me how to do buffer overflows and gave me so many attempts at doing them that I got the basics down and felt like I conquered a huge undertaking. Buffer Overflows can be a lot to take in and work on and with Buffer Overflow Prep I was able to overcome the fear and learn to have fun with them.
That is why I recommend Buffer Overflow Prep and why it is one of my favorite TryHackMe rooms. It gave me the tools and knowledge needed to learn how to perform a Buffer Overflow and how to transfer the knowledge to other rooms and exams.
I know someone else out there is afraid to dive into Buffer Overflows and if that someone is you, try this room and watch the video Tib3rius made for this room. Stack Based Buffer Overflow Prep.
4. Linux/Windows Priv Esc

I’m cheating here, but I’m going to combine Linux and Windows Privilege Escalation rooms together for the number four spot.
Created by Tib3rius, these rooms are geared towards teaching some of the most important techniques that are required to go anywhere in this field. A short list of what you will learn are
Linux:
- Weak File Permissions
- Cron
- SUID
- NFS
- Kernel Exploits
Windows:
- Registry
- Scheduled Tasks
- Token Impersionation
- Service Exploits
This is a short and sweet description, but nothing can really be said other than if you want to be a penetration tester / red teamer than these two rooms are essential.
A small bonus I found helpful was to take the Udemy courses by Tib3rius as I did these rooms. The Udemy videos and TryHackMe rooms do an excellent job complimenting each other.
Links to the Udemy courses can be found here.
3. Biohazard

For those that do not know this about me, I love the Resident Evil games and Biohazard does a phenomenal job capturing the feel of playing a RE game while hacking.
It is one of the more “video game” like rooms in both name and execution. You are tasked to collect flags and discovered hidden files, folders, pages, keys, images, and anything else scatted on the box to complete this room. It is simply a blast to play.
It can be difficult and at times I would get stuck and get a bit frustrated, but that emulated the feel of the RE games so much that I had to smile. Come prepared to think outside the box and don’t forget about that ever important source code to get by.
Not beginner friendly and it is one of the paid sub rooms, but if you haven’t played this room and love the Resident Evil franchise then I highly recommend this room.
Even if you do not play the Resident Evil games I still recommend this room. It is nothing but a crazy, fun puzzle.
2. Mr. Robot

This room is simply charming and fun to play with. Similar to Vulnversity it comes with a video guide by Darkstar to help hold your hand and provide though process and techniques used to help get through the room.
This room is marked as medium difficulty, but I would say it is one of the best rooms to transition someone that has been doing easy rooms and wants to step their game up and try the medium difficulty rooms.
If you are looking for a fun room to step up your game and have fun. I cannot recommend Mr. Robot enough. I was smiling and having such a blast hacking this box. It is easily one of the best TryHackMe has to offer.
1. Internal

My absolute favorite TryHackMe room is Internal, created by Joe Helle aka TheMayor. I love rooms that are more geared towards real world challenges and are exactly like what a penetration tester would see in the wild. This room was also designed to help prepare you for the eLearnsecurity eCPPT.
Just like in a real penetration test you are given a scope of work and are encouraged to create a report. Once you have completed reading the briefing and start the machine you need to obtain a user.txt flag and root.txt flag.
This room is rather difficult and not for beginners. It is a fantastic room to see where your skills are at and what needs improvement. Do not use a guide for this room. Internal is a room that if you can complete without help can really demonstrate to yourself that you have a good skill set when it comes to penetration testing.
TheMayor truly knocked it out of the park creating this room and even though I have completed it I will often come back to again and again just like one of my favorite video games. The amount of detail put into this room is excellent and I cannot recommend it enough to put your skills to the test.
In Conclusion: I hope this blog has provided some insight for those looking for suggestions for rooms on TryHackMe. TryHackMe has been a huge part in my growth as a Penetration Tester. The overwall number of rooms is huge and it can be a bit much to find the next room to do and I hope this helps someone!
If it does, feel free to send me a message on Twitter and let me know!